Recursion and Sorting Algorithms
Contents
Recursion
- function calls itself.
- Boil down a big problem to smaller ones.
- Implementation:
- Base case: smallest problem to solve.
- Subproblem: smaller size of the pre-problem. (when we have the result of the subproblem, the what you need to do to solve the pre-problem based on subproblem)
- Recursive rule: how to make the problem smaller. (if we can solve the problem but with a smaller size, then what is left to do for the current problem size)
Fibonacci
|
|
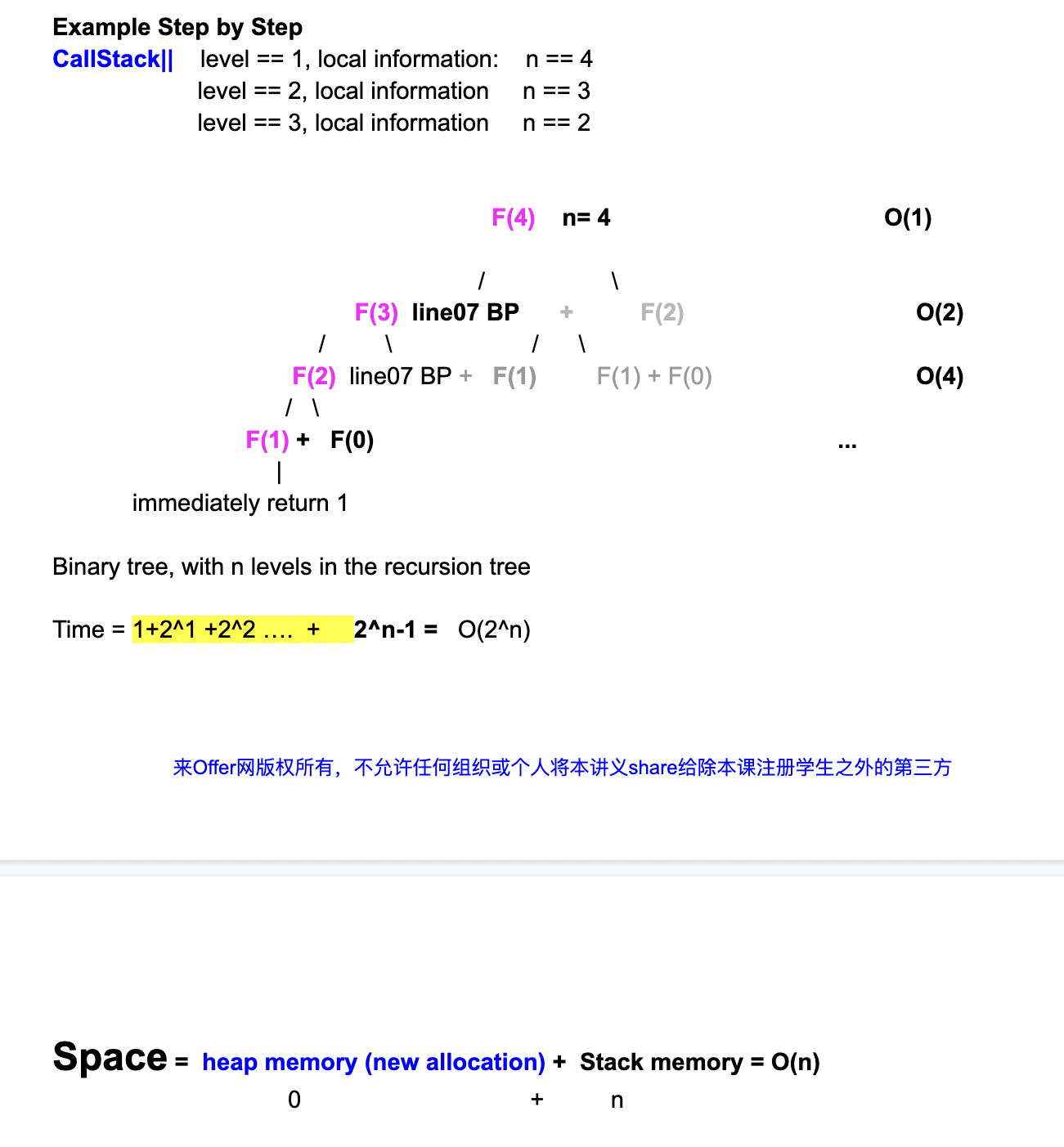
How to analyze time and space complexity
- Time Complexity: The sum of time complexity of all nodes in the recursive tree.
- Space Complexity: The sum of space complexity of all nodes on a path from top to bottom.
- Space Complexity =
heap
memory +stack
memory - Call Stack: it is a global accessible resource that can tell what happened before each break point in each level.
a to the power of b
|
|
Sorting Algorithm
Code Explanation Tips
- Document your assumption.
- Explain your approach and how you intend to solve the problem.
- Provide code comments where applicable
- Explain the big-O run time complexity of your solution, justify your answer.
- Identify any additional data structures you used, and justify why you used them.
- Only provide your best answer to each part of the question.
- When explaining an algorithm you wrote, you should explain in a modular step, like what is a code block doing.
Selection Sort
|
|
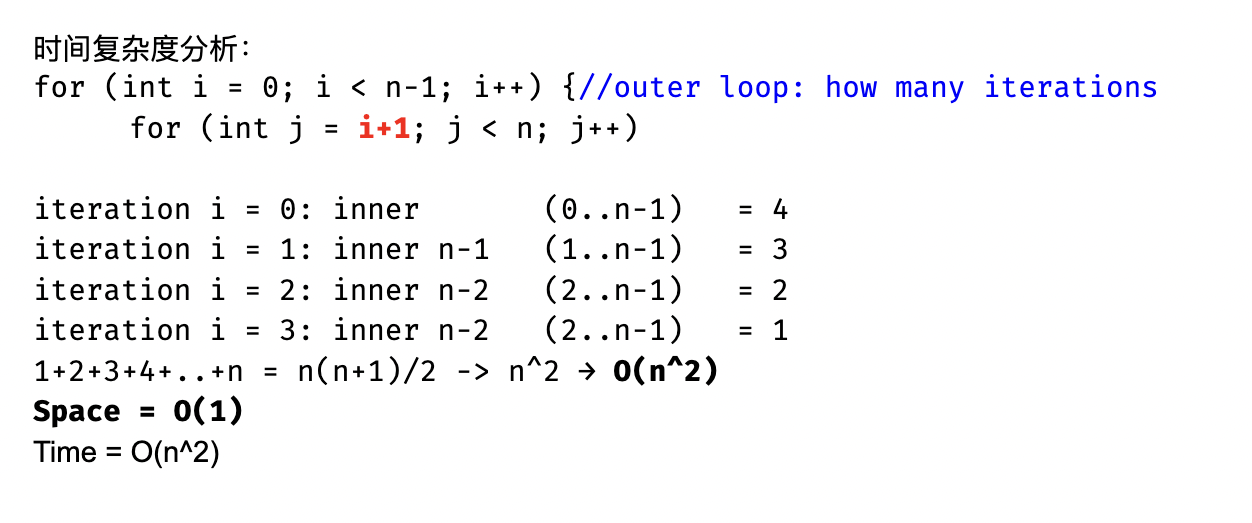
Merge Sort
how to merge two arrays
We get the smaller one stored first and then move this one’s index, and then repeat this process.
Also, we need a temporary space to buffer the result. like if the array size is 8, then the temporary space size is 8 (int[] space = new int[8];
)
|
|
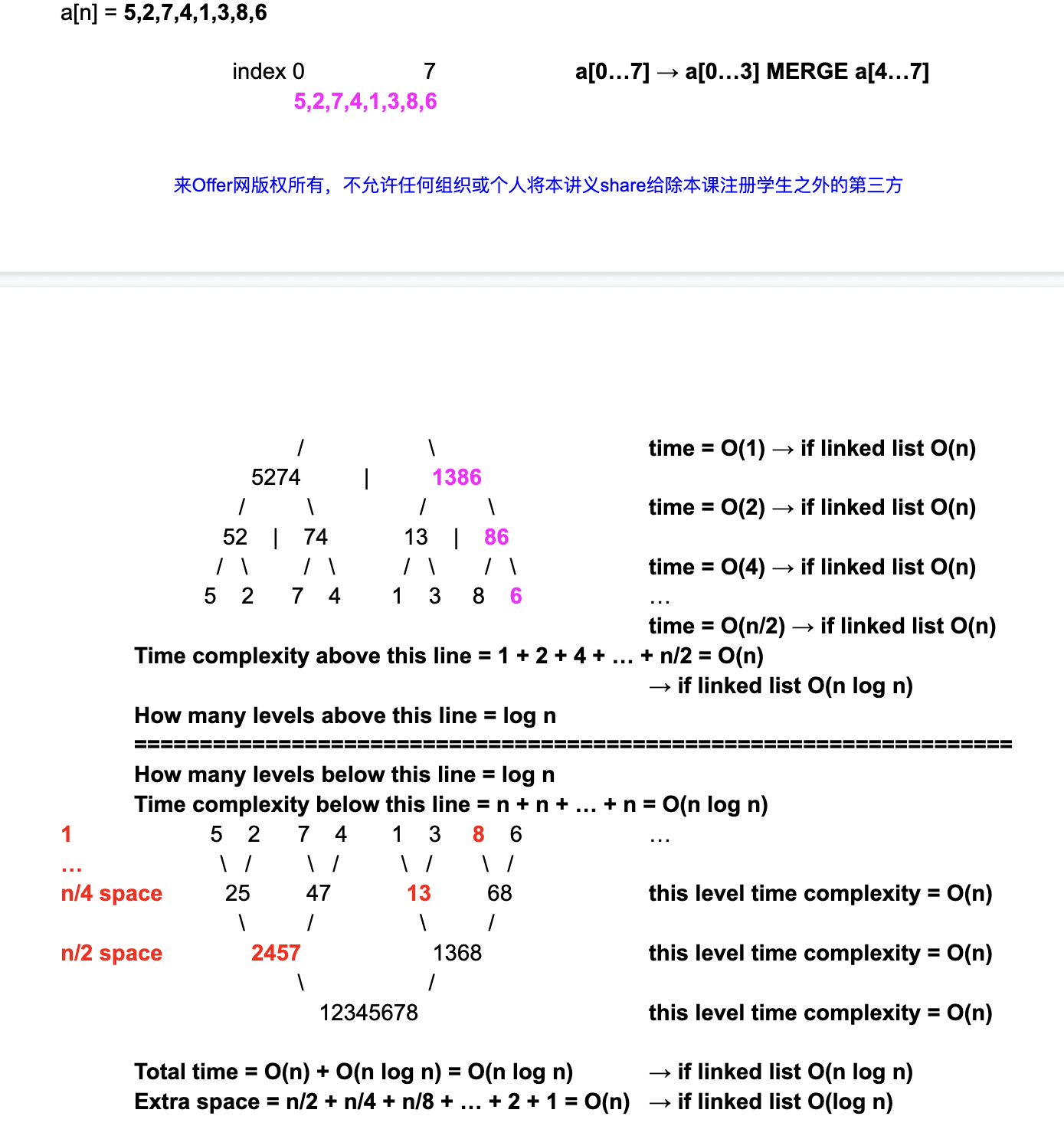